PHP
Traces | Metrics | App Logs | Custom Logs | Profiling |
---|---|---|---|---|
✅ | ✅ | ✅ | ✖ | ✖ |
This guide walks you through setting up Application Performance Monitoring (APM) on a PHP application. These instructions can also be found on the Installation page in your Middleware Account. View example code here.
The CakePHP web framework is not currently supported by the PHP APM.
Currently, PHP APM is only supported on Debian-based distributions.
Prerequisites
Infra Agent: Infrastructure Agent (Infra Agent). To install the Infra Agent, see our Installation Guide.
PHP:
PHP version 8.0
or above. Verify withphp -v
.Composer: Ensure you have the Composer dependency manager installed to install our APM.
PECL: PECL is required to install the appropriate extension.
Vanilla PHP
Setup
Before installing any Middleware APM package for PHP, the auto-instrumentation extension must be setup beforehand.
Install the Auto-Instrumentation Extension
Shell
sudo pecl install channel://pecl.php.net/opentelemetry-1.0.3
Add Extension to php.ini
index.php
[opentelemetry] extension=opentelemetry.so
The php.ini
file can be found using the following commands based on your Linux distribution:
Shell
sudo nano /etc/php/<php_version>/cli/php.ini
Shell
sudo nano /etc/php.ini
Shell
sudo nano /etc/php/php.ini
Shell
sudo nano /etc/php/<php_version>/apache2/php.ini
Verify Installation
Verify the extension is installed and enabled.
Shell
php -m | grep opentelemetry
Ensure you have followed the steps in the Setup section before continuing
Install the APM Package
To install the Middleware APM package, run the following commands:
Shell
composer update composer require middleware/agent-apm-php
Import the Tracker
Add following code to the entry point of your application, and as the first import:
index.php
require 'vendor/autoload.php'; use Middleware\AgentApmPhp\MwTracker;
[Optional] Container Variables
Docker
For use with Docker, add the following environment variable to your application.
Shell
MW_AGENT_SERVICE=<DOCKER_BRIDGE_GATEWAY_ADDRESS>
The DOCKER_BRIDGE_GATEWAY_ADDRESS
is the IP address of the gateway between the Docker host and bridge network. This is 172.17.0.1
by default. Learn more about Docker bridge networking here
Kubernetes
For use with Kubernetes, first ensure the Middleware agent is installed in your cluster by using the following command:
Shell
kubectl get service --all-namespaces | grep mw-service
Add the following environment variable to your application deployment YAML file:
Shell
MW_AGENT_SERVICE=mw-service.mw-agent-ns.svc.cluster.local
Implement APM Collector
Start a tracing scope right before your main application code:
index.php
$tracker = new MwTracker('<PROJECT-NAME>', '<SERVICE-NAME>'); $tracker->preTrack();
Define hooks with class and function names beneath the tracing scope:
index.php
$tracker->registerHook('<CLASS-NAME-1>', '<FUNCTION-NAME-1>', [ 'custom.attr1' => 'value1', 'custom.attr2' => 'value2', ]); $tracker->registerHook('<CLASS-NAME-2>', '<FUNCTION-NAME-2>');
End the tracing scope:
index.php
$tracker->postTrack();
Enable Logging
To ingest custom logs, use the following functions based on desired log severity levels.
index.php
$tracker->warn("this is warning log."); $tracker->error("this is error log."); $tracker->info("this is info log."); $tracker->debug("this is debug log.");
Wordpress
Get the script
Change your directory to your wordpress installation directory (i.e. /var/www/localhost/htdocs)
and run following command:
curl -O https://install.middleware.io/apm/php/wp-instrument.php
You can also download the script by clicking here.
Installation
Run the script with administrator or root privileges:
sudo -E MW_SERVICE_NAME=<your-service-name> php wp-instrument.php
Here, MW_SERVICE_NAME
is optional, default service name will be service-<process id>
.
Docker
For use with Docker, add the following environment variable to installation script.
Shell
sudo -E MW_TARGET=<DOCKER_BRIDGE_GATEWAY_ADDRESS> php wp-instrument.php
The DOCKER_BRIDGE_GATEWAY_ADDRESS
is the IP address of the gateway between the Docker host and bridge network. This is 172.17.0.1
by default. Learn more about Docker bridge networking here
Laravel
Laravel version 8.x
or higher required
Installation
Change your directory to laravel project's root directory, and run following commands to install the apm:
Shell
curl -O https://install.middleware.io/apm/php/laravel-instrument.php && sudo php laravel-instrument.php install
This script installs necessary components and configures APM easily.
Usage
This script has a install
command which Verifies prerequisites, Installs and configures required extensions and packages for instrumentation.
You can also download the script by clicking here.
Tracing
Traces are automatically enabled.
Metrics
Currently, support for metrics is limited
To enable trace-related metrics Add the LaravelApmServiceProvider
to the providers
array in config/app.php
:
app.php
'providers' => [ // ... Middleware\LaravelApm\LaravelApmServiceProvider::class, ],
and add the MetricsMiddleware
class to the $middleware
array in app/Http/Kernel.php
. If you have enabled tracing, be sure to add this after the tracing class:
Kernel.php
protected $middleware = [ // ... \Middleware\LaravelApm\Middleware\MetricsMiddleware::class, ];
Logging
The package automatically integrates with Laravel's logging system to send logs to Middleware. Ensure the correct log driver and settings are configured in your Laravel app, and the package will automatically handle log capture and export.
Run your app
Set following variables in your environment and restart your app to instrument your app:
env
OTEL_PHP_AUTOLOAD_ENABLED=true OTEL_SERVICE_NAME=your-service-name OTEL_EXPORTER_OTLP_ENDPOINT=http://localhost:9320
To correlate frontend traces with backend traces, add following environment variable:
env
OTEL_PROPAGATORS=baggage,tracecontext,b3multi
Docker
For use with Docker, add the following environment variable to installation script.
Shell
sudo -E MW_TARGET=<DOCKER_BRIDGE_GATEWAY_ADDRESS> php laravel-instrument.php run php artisan
The DOCKER_BRIDGE_GATEWAY_ADDRESS
is the IP address of the gateway between the Docker host and bridge network. This is 172.17.0.1
by default. Learn more about Docker bridge networking here
Azure Pipelines
The Azure Pipelines continuous integration and continuous delivery (CI/CD) infrastructure helps you build, deploy, and test your PHP projects. For information on setting up your PHP project with Azure Pipelines and Azure DevOps Services, learn more here.
Step 1: Configure OpenTelemetry Extension
Add the following code to your .ini
file located in the /home/html/ini
directory of your Azure account:
extension.ini
extension=opentelemetry.so
Step 2: Add Configuration to Azure
Navigate to Application Settings in your Azure PHP project account and select New application setting
. Add the following Name and Value to the New application setting
:
Azure
Name: PHP_INI_SCAN_DIR Value: /home/html/ini
Your configuration should look like the following:
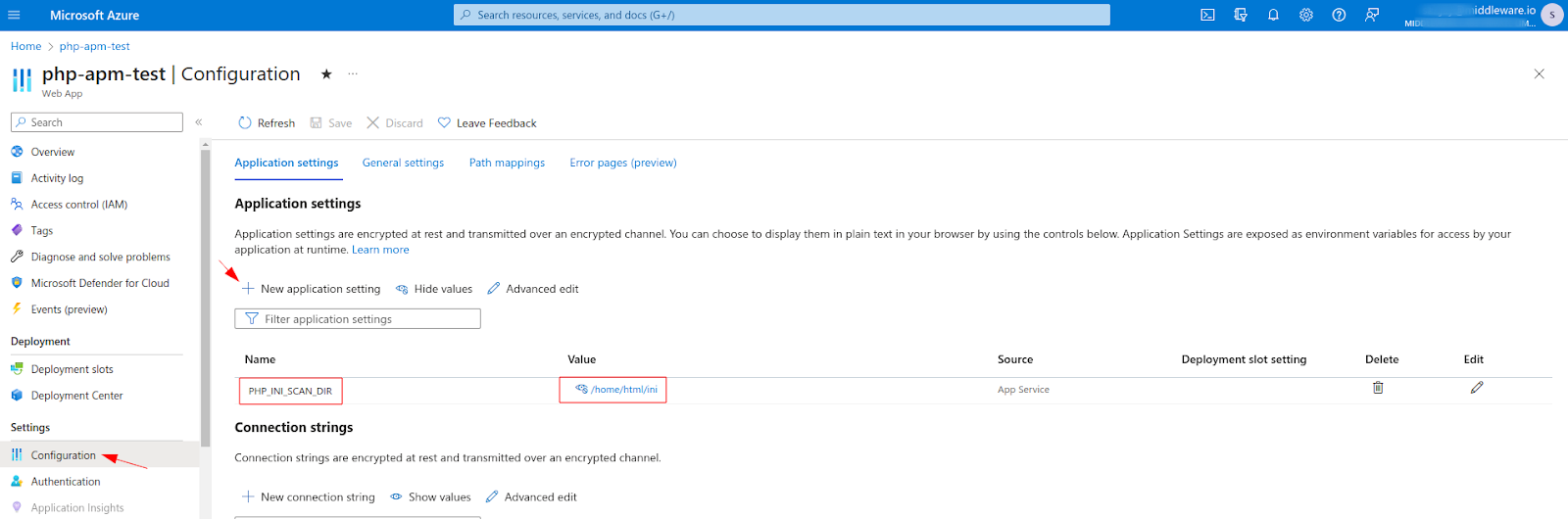
Step 3: Create Your Build Pipelines
Update your build pipeline by adding the following code to the azure-pipelines.yml
file:
The below build pipeline includes installation steps for Opentelemetry
and the middleware/agent-apm-php
.yaml
trigger: - master variables: # Azure Resource Manager connection created during pipeline creation azureSubscription: 'XXXXXXXXXXXXXXXXXXXX' # Web app name webAppName: 'php-apm-test'
Step 4: Check Your Pipeline
The PHP Agent is now set up to execute a CI/CD approach, following the build pipeline configuration, whenever new code is pushed to the production environment.
Your output should look like the following:
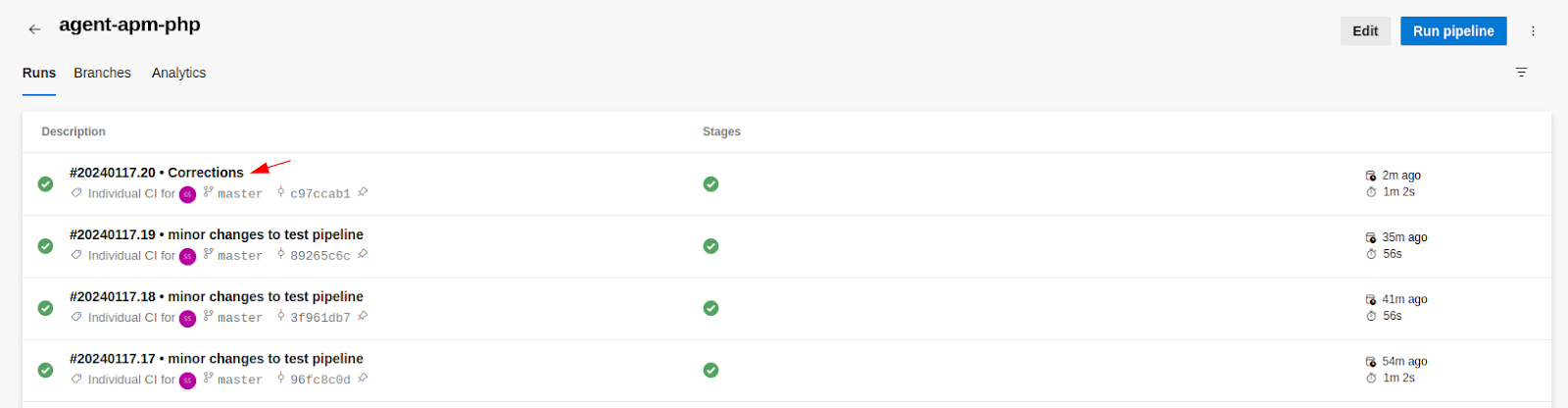
Troubleshooting
Below we cover a few common errors and their solutions.
pecl: command not found error
If you receive a pecl: command not found error
, run the following command:
Shell
sudo apt-get update apt-get install php-pear php8.1-dev
Broken Dependency Issue
If you receive any broken dependency issues, including errors like libpcre2-dev : Depends: libpcre2-8-0 / libpcre2-16-0 / libpcre2-32-0
, run the following command:
Shell
sudo apt --fix-broken install
ERROR: ‘phpize’ failed error
If you receive an ERROR: ‘phpize’ failed error
, run the following commands:
Shell
sudo apt-get update sudo apt-get install php8.1-dev sudo apt-get update sudo pecl channel-update pecl.php.net
Continuous Profiling
Continuous profiling captures real-time performance insights from your application to enable rapid identification of resource allocation, bottlenecks, and more.
Continuous Profiling for the PHP APM is not yet available; reach out to our support team if you'd like more information. Navigate to the Continuous Profiling section to learn more about using Continuous Profiling with our other APMs.
Need assistance or want to learn more about Middleware? Contact our support team in Slack.