.NET
Traces | Metrics | App Logs | Custom Logs | Profiling |
---|---|---|---|---|
✅ | ✅ | ✖ | ✅ | ✅ |
This guide walks you through setting up Application Performance Monitoring (APM) on a .NET application. These instructions can also be found on the Installation page in your Middleware Account. View example code here.
Prerequisites
1 Infra Agent
To install the Infra Agent, see our Installation Guide.
2 .NET Version
.NET version 6+
. Check your .NET version with the following command:
dotnet --version
Install
Step 1: Setup Middleware .NET Project
Download the latest Middleware.dll and add the following code in the .csproj
file.
<ItemGroup> <Reference Include="Middleware"> <HintPath>path\to\Middleware.dll</HintPath> </Reference> </ItemGroup>
Step 2: Install .NET
APM Package
Run the following command in your terminal
curl -sSfL https://install.middleware.io/apm/dotnet/v1.0.0-rc.1/scripts/mw-dotnet-auto-install.sh -O
Install core files
sh ./mw-dotnet-auto-install.sh rm -f mw-dotnet-auto-install.sh
Enable execution for the instrumentation script
chmod +x $HOME/.mw-dotnet-auto/instrument.sh
Execute the following command run your .NET application:
MW_API_KEY="<MW_API_KEY>" \ . $HOME/.mw-dotnet-auto/instrument.sh && \ OTEL_SERVICE_NAME="{APM-SERVICE-NAME}" \ dotnet path/to/YourApplication.dll
Run the following command in powershell your terminal
$install = Join-Path $env:temp "mw-dotnet-auto-install-setup.ps1" Invoke-WebRequest -Uri "https://install.middleware.io/apm/dotnet/v1.0.0-rc.1/scripts/mw-dotnet-auto-install-setup.ps1" -OutFile $install -UseBasicParsing
Execute the script
. $install
Register the .NET service name
Register-OpenTelemetryForCurrentSession -OTelServiceName "{APM-SERVICE-NAME}"
Run your application with instrumentation.
.\YourNetApp.exe
If you are running your .NET app as a container, you can include all this steps in your Dockerfile as mentioned below
RUN apt-get update && apt-get install -y curl unzip RUN curl -sSfL https://install.middleware.io/apm/dotnet/v1.0.0-rc.1/scripts/mw-dotnet-auto-install.sh -O RUN bash mw-dotnet-auto-install.sh RUN rm -rf mw-dotnet-auto-install.sh RUN chmod +x $HOME/.mw-dotnet-auto/instrument.sh ........... CMD . $HOME/.mw-dotnet-auto/instrument.sh && dotnet Mw-WebApplication.dll
Add the following environment variables to your container:
MW_AGENT_SERVICE=172.17.0.1 MW_API_KEY="<MW_API_KEY>"
The DOCKER_BRIDGE_GATEWAY_ADDRESS
is the IP address of the gateway between the Docker host and bridge network. This is 172.17.0.1
by default. Learn more about Docker bridge networking here
Specify your HTTP ports and URL with the following:
This only applies to .NET projects using the ASP framework.
Dockerfile
ASPNETCORE_HTTP_PORTS=5000 ASPNETCORE_URLS=http://0.0.0.0:5000
If you are running your .NET app as a Kubernetes container, you can include all this steps in your Dockerfile as mentioned below
RUN apt-get update && apt-get install -y curl unzip RUN curl -sSfL https://install.middleware.io/apm/dotnet/v1.0.0-rc.1/scripts/mw-dotnet-auto-install.sh -O RUN bash mw-dotnet-auto-install.sh RUN rm -rf mw-dotnet-auto-install.sh RUN chmod +x $HOME/.mw-dotnet-auto/instrument.sh ........... CMD . $HOME/.mw-dotnet-auto/instrument.sh && dotnet Mw-WebApplication.dll
Add the following environment variables to your container:
MW_AGENT_SERVICE=mw-service.mw-agent-ns.svc.cluster.local MW_API_KEY="<MW_API_KEY>"
The default namespace for running the Middleware agent is mw-service.mw-agent-ns.svc.cluster.local
.
Sending Custom Data [Optional]
Enable Custom Logs
Add the following functions:
using Middleware.Logger; ... Logger.Info("This is info log"); Logger.Warning("This is info log"); Logger.Debug("This is info log"); Logger.Error(<Object of Exception>);
Add packages to YourApp.csproj
file
<Project Sdk="Microsoft.NET.Sdk.Web"> ... <ItemGroup> <PackageReference Include="OpenTelemetry" Version="1.5.1" /> <PackageReference Include="OpenTelemetry.Exporter.Console" Version="1.5.1" /> </ItemGroup> ... </Project>
Enable Logs in your project.cs
file
index.cs
using OpenTelemetry.Logs; using OpenTelemetry.Resources; ... var builder = WebApplication.CreateBuilder(args); builder.Logging.AddOpenTelemetry(options => { options.AddConsoleExporter(); });
Continuous Profiling
Continuous profiling captures real-time performance insights from your application to enable rapid identification of resource allocation, bottlenecks, and more. Navigate to the Continuous Profiling section to learn more about using Continuous Profiling with the .NET APM.
.NET Nuget Packge APM Configuration
Traces | Metrics | App Logs | Custom Logs | Profiling |
---|---|---|---|---|
✅ | ✅ | ✖ | ✅ | ✖ |
Step 1: Install the Required Package
Add the Middleware package to your .NET project by running the following command using CLI:
dotnet add package MW.APM --version 1.1.1
OR
If you are using Visual Studio IDE in Windows, then you can install it by going to below given path.
PATH - Tools > Nuget Package Manager > Manage Nuget Packages for Solutions > Browse
Now, search for MW.APM and Install the nuget package.
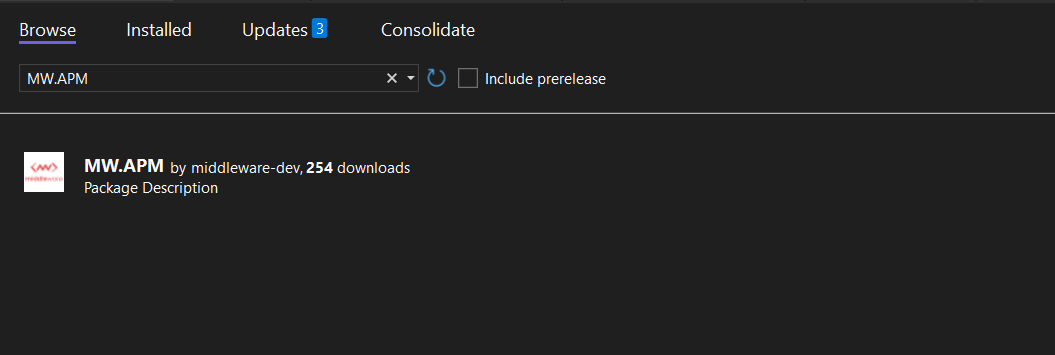
Step 2: Add Code Configuration
Add the following code to your Program.cs
file:
Program.cs
var configuration = new ConfigurationBuilder() .SetBasePath(Directory.GetCurrentDirectory()) .AddJsonFile("appsettings.json", optional: false, reloadOnChange: true) .AddEnvironmentVariables() .Build(); builder.Services.ConfigureMWInstrumentation(configuration); builder.Logging.AddConfiguration(configuration.GetSection("Logging")); builder.Logging.AddConsole();
Step 3: Configure Your Middleware Account Information
Add the following to your appsettings.json
file:
"MW": { "ApiKey": "<MW_API_KEY>", "TargetURL": "https://<MW_UID>.middleware.io:443", "ServiceName": "<service-name>", "ProjectName": "<project-name>", "ConsoleExporter": "true", "ExcludeLinks": "[\"https://localhost:3000/health\"]", "ApmCollectMetrics": "true", "ApmCollectTraces": "true", "ApmCollectLogs": "true"
appsetting.json Config Attribute | Description |
---|---|
ApiKey | MW API Key of your account. It is required field. |
TargetURL | MW Target URL of your account. It is required field. |
ServiceName | If this key not provided then default value unknown_service:dotnet. |
ProjectName | If this key not provided then default value blank. |
ConsoleExporter | This key useful when user wants to debug APM. If this key not provided then default value false . |
ExcludeLinks | If user wants to exclude some APIs generated using Http client from tracing. If this key not provided then default value empty list. |
ApmCollectMetrics | Key for enabling Metrics Instrumentation. If this key not provided then default value true . |
ApmCollectTraces | Key for enabling Traces Instrumentation. If this key not provided then default value true . |
ApmCollectLogs | Key for enabling Logs Instrumentation. If this key not provided then default value true . |
This is a one-time configuration. After these configuration changes are made, each time the .NET app will run, the .NET instrumentation will also run.
Need assistance or want to learn more about Middleware? Contact our support team at [email protected].